In the world of programming, automating repetitive tasks is a key skill that can save time and reduce errors. In this blog post, we’ll explore two common scenarios: comparing CSV files and replacing text in a file, using Python.
Comparing CSV Files
Objective: Given two CSV files, we want to compare values based on a common column and identify any differences: use the first CSV as the anchor/dictionary, search each name in the first column, and if it exists in both CSV files, compare the value, if they are different, create a new CSV to list the differences.
CSV format:
name,value
IP_10.0.0.1,10.0.0.1
Host_10.0.0.2,10.0.0.2
Solution:
We can achieve this using Python. Here’s a simple script that reads two CSV files and creates a new CSV file listing the differences:
import csv
def compare_csv_files(file1_path, file2_path, output_path):
# Read the content of the first CSV file into a dictionary
data_dict = {}
with open(file1_path, 'r') as file1:
reader1 = csv.reader(file1)
for row in reader1:
if row:
name = row[0]
value = row[1]
data_dict[name] = value
# Compare the second CSV file with the data from the first file
differences = []
with open(file2_path, 'r') as file2:
reader2 = csv.reader(file2)
for row in reader2:
if row:
name = row[0]
value = row[1] if len(row) > 1 else None
if name in data_dict:
if value != data_dict[name]:
differences.append([name, data_dict[name], value])
# Write the differences to a new CSV file
with open(output_path, 'w', newline='') as output_file:
writer = csv.writer(output_file)
writer.writerow(['Name', 'Value in File1', 'Value in File2'])
writer.writerows(differences)
# Example usage
compare_csv_files('file1.csv', 'file2.csv', 'differences.csv')
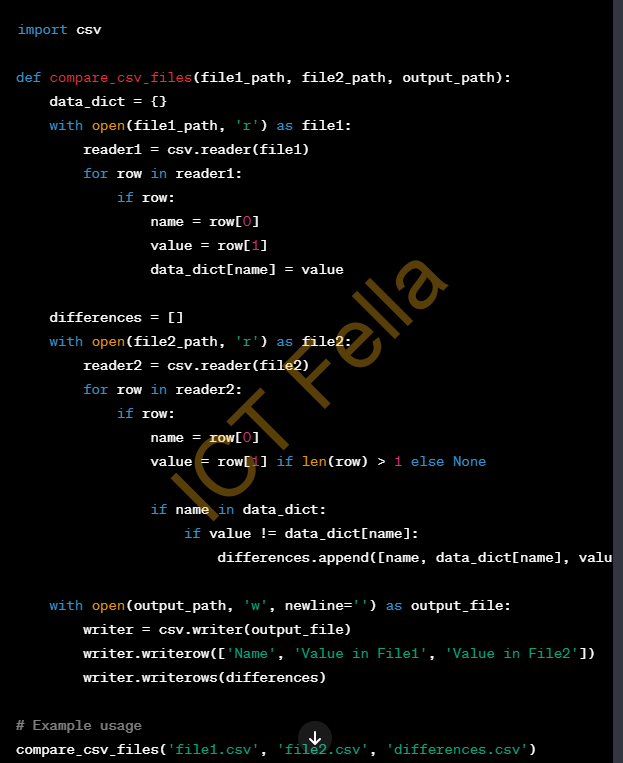
Text Replacement in a File
Objective: Given a text file and a CSV file with find and replace mappings, we want to replace specific values in the text file.
Solution:
Here’s a Python script that accomplishes this task:
import csv
def find_replace_text(input_text_file, csv_file, output_text_file):
find_replace_mappings = {}
with open(csv_file, 'r') as csv_file:
reader = csv.DictReader(csv_file)
for row in reader:
find_replace_mappings[row['find']] = row['replace']
with open(input_text_file, 'r') as input_file:
input_text = input_file.read()
for find_str, replace_str in find_replace_mappings.items():
input_text = input_text.replace(find_str, replace_str)
with open(output_text_file, 'w') as output_file:
output_file.write(input_text)
# Example usage
find_replace_text('input.txt', 'find_replace_mapping.csv', 'output.txt')
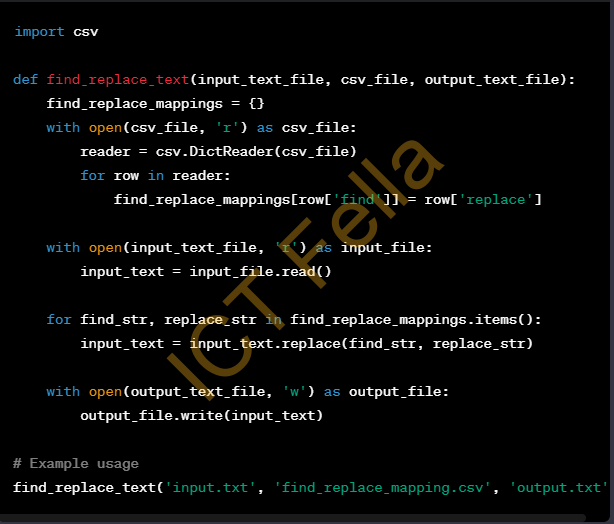
This script reads find and replace mappings from a CSV file and replaces the specified values in the input text file, creating a new output text file.
These two scripts can be customized and integrated into your workflow to automate these tasks efficiently. Feel free to adapt them to your specific requirements!
Happy coding!